I added a failing test to my system with 2 issues:
One being that the new value didn’t get set to “”, and the second problem being that it did not consider Null any different than the new random value. Two problems we can tackle in our next episode.
TDD – 033 article
And the test code is as follows:
Private Sub FormToAudit_BeforeUpdate(Cancel As Integer)
If FormToAudit.TestText.OldValue <> FormToAudit.TestText.Value Then
pListOfChanges.Add FormToAudit.TestText.Value
End If
End Sub
I guess we also need to fix the issue of passing the wrong value in the other function:
Private Sub ChangeTestText(Optional NewValue As Variant = "")
If NewValue = "" Then
Randomize Timer
NewForm.TestText = "New Thing " & Rnd()
Else
NewForm.TestText = NewValue
End If
NewForm.Dirty = False
End Sub
So we can see if I specifically pass my helper function ChangeTestText and Empty String it creates default randomized output. I’m going to solve this by splitting this into two functions, one that requires the variable and one that takes no variable.
Voila:
Private Sub ChangeTestText(NewValue As Variant)
NewForm.TestText = NewValue
NewForm.Dirty = False
End Sub
Private Sub ChangeTestTextToRandomString()
Randomize Timer
ChangeTestText "New Thing " & Rnd()
End Sub
And now I need to update my original tests that don’t use a parameter to use the new function name which is just these two tests:
'@TestMethod("Count Changes")
Private Sub WhenOneFieldIsChangedThenReturnSingleListOfChanges()
Dim testFormAuditor As FormAuditor
Dim testCollection As New Collection
Set testFormAuditor = New FormAuditor
ChangeTestTextToRandomString
Set testCollection = testFormAuditor.ListOfChanges
Assert.AreEqual CLng(1), testCollection.Count
End Sub
'@TestMethod("Count Changes")
Private Sub WhenTwoFieldsAreChangedThenReturnTwoEntryListOfChanges()
Dim testFormAuditor As FormAuditor
Dim testCollection As Collection
Set testFormAuditor = New FormAuditor
ChangeTestTextToRandomString
ChangeTestTextToRandomString
Set testCollection = testFormAuditor.ListOfChanges
Assert.AreEqual CLng(2), testCollection.Count
End Sub
Ok, I will run the tests to make sure my original tests are still passing while my new test should still be failing. And I’m right, that’s what’s happening:
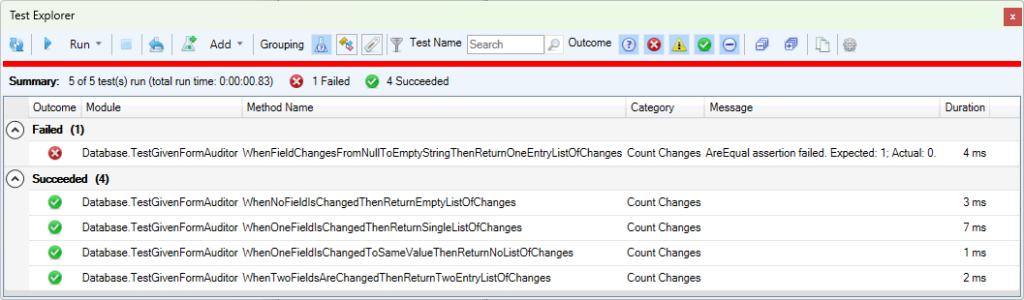
Ok, now I’m passing the right data using the test, but now there is still a problem with the null to string comparison.
I think I can quickly solve that by using If or Select Case statements to determine when the new or old value (or both) are null and make our logic conclusions that way. Ugh, it was quick but really ugly!
Private Sub FormToAudit_BeforeUpdate(Cancel As Integer)
Dim OldValue As Variant, NewValue As Variant, AddChange As Boolean
OldValue = FormToAudit.TestText.OldValue
NewValue = FormToAudit.TestText.Value
If IsNull(OldValue) And Not IsNull(NewValue) Then
AddChange = True
ElseIf Not IsNull(OldValue) And IsNull(NewValue) Then
AddChange = True
ElseIf IsNull(OldValue) And IsNull(NewValue) Then
AddChange = False
Else
If FormToAudit.TestText.OldValue <> FormToAudit.TestText.Value Then
AddChange = True
End If
End If
If AddChange Then
pListOfChanges.Add FormToAudit.TestText.Value
End If
End Sub
And my test now passes:
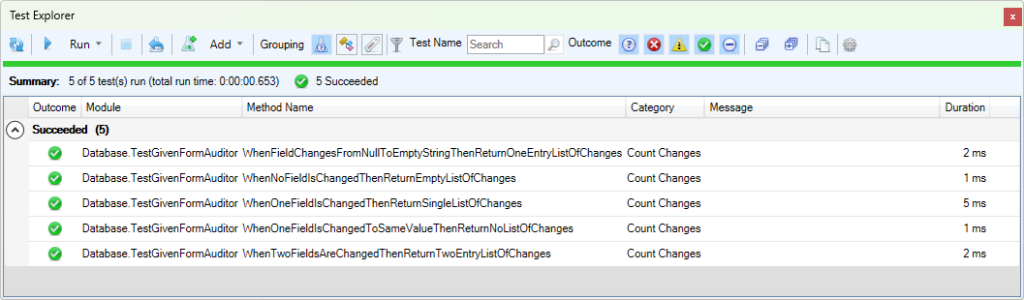
Thankfully, our next phase is the refactor phase. I know something I’m about to refactor! Tomorrow…