Ok, opened up the FormAuditor database and ran the tests. Here’s where I’m at:
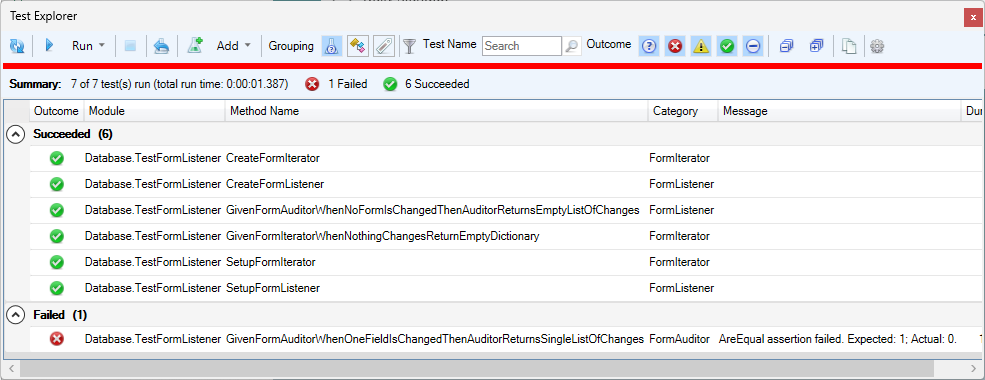
That test name is a mouthful! GivenFormAuditorWhenOneFieldIsChangedThenAuditorReturnsSingleListOfChanges
Given: FormAuditor
When: One Field Is Changed
Then: AuditorReturnsSingleListOfChanges
So my test again looks like this:
And yes, I think that name is a bit too long. I know I was defending my long names, but that one is sooo long it’s rather hard to comprehend without breaking it out into it’s components.
Anyway, renaming tests is something to do when refactoring, not when making a test pass, so let’s make this test pass!
I may need to add a little more code to the test eventually, but let’s see if I can make it work as is.
First I need to configure the FormAuditor to hook into the BeforeUpdate event of the form, so let’s give that a shot.
I’m adding a Private form variable to the FormAuditor class using the WithEvents keyword:
I’ve already instantiated the form we are going to use before the test runs, so I should just be able to connect to it when making the FormAuditor instance by using the Class_Initialize event.
That should work for initialization. Now I’ll create the BeforeUpdate event in the FormAuditor class:
And for that to work, I’ll need to make a Private class variable for the dictionary and update the ListOfChanges public property to return this internal variable. Here is the full FormAuditor class now:
I think this might pass, so I’ll make a go of it. Let’s see what happens:
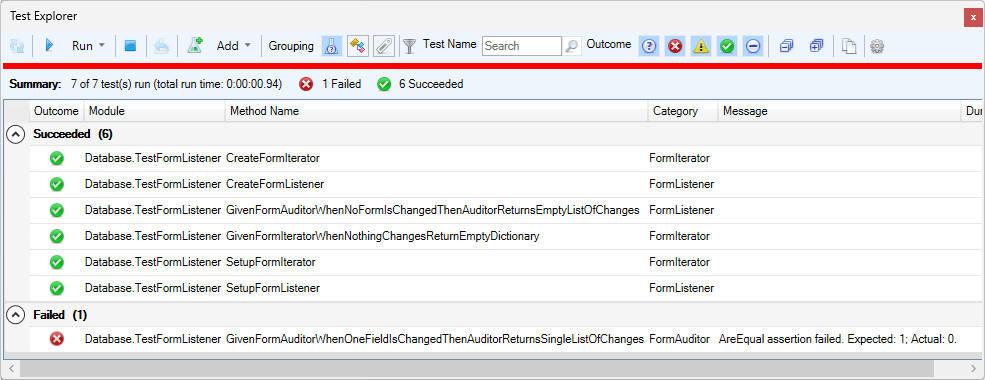
Bummer, it didn’t pass. It’s not seeing a dictionary with one element.
I’ll try setting a break point in the BeforeUpdate FormAuditor event. I suspect it’s not firing. That didn’t help, so I set a break point in the Test instead and walked through it. I thought that the class initialize method would run when I used the New keyword when Dimming the testFormAuditor varaible, but as I stepped through the test code, the class initialize function didn’t run until I called testFormAuditor.ListOfChanges. By that time we had already changed the form. So, I’m going to change the test slightly to Set the testFormAuditor = New FormAuditor and see if that will run the class initialize method.
Here is the new code for the test:
I’ll see if that works next time.