The latest test is failing due to a compiler error:
I don’t have the ListOfChanges property in the class yet. So I will add it. Here is my first update to the FormAuditor class:
Now, run the tests and… I got another compiler error for the misspelling of Dicctionary above (note the 2 c’s). After fixing that, the test runs but gets the wrong type of return value:
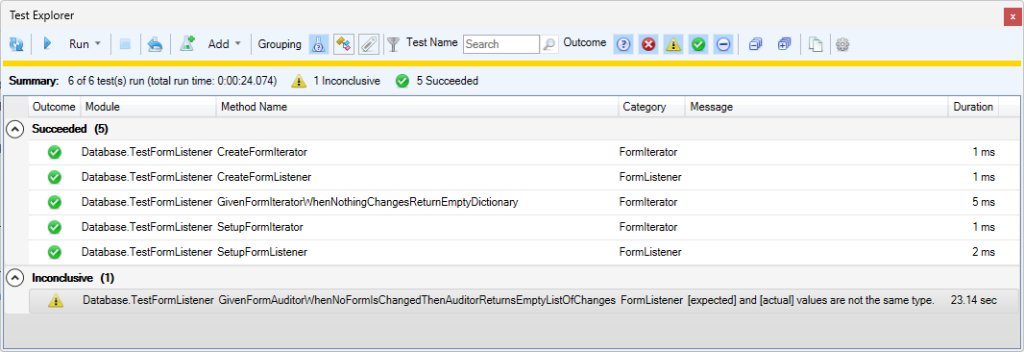
What’s happening here is that I am comparing a literal zero with the result of the Dictionary Count Property. I have to cast the literal zero to be the same type of number as the number returned by the Count property.
According to the documentation on Microsoft’s Learn site the Count property returns a number of type Long: Count property (Dictionary object) | Microsoft Learn
Here is the new FormAuditor class with the typo corrected so it will compile:
And here is the updated test to compare the numbers both as Longs:
And now the test passes. Woo-hoo!
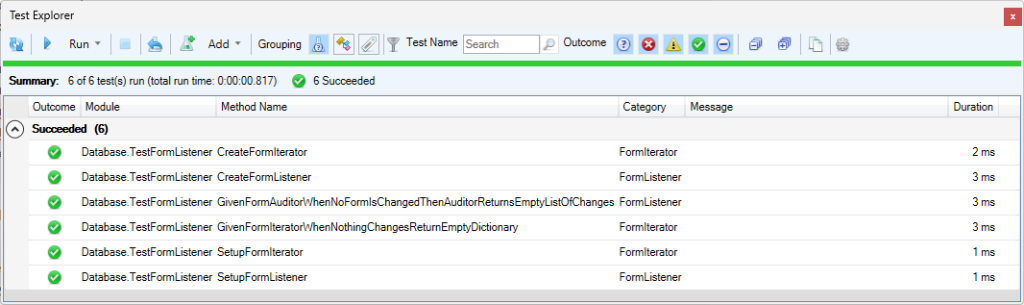
Ok, great. Now I understand one of the principles of simple testing when it comes to numbers is to first test the zero condition, then a one condition, and finally a many condition (where typically two is many) So I forego my Refactoring at this stage in favor of putting together the test of ONE change. So if there is ONE change to the form, I should get a dictionary with ONE entry. Not going to worry about what I’m going to put in the dictionary entry yet, but Let’s see if I can get the test written:
All right, that should definitely fail, even if it compiles…
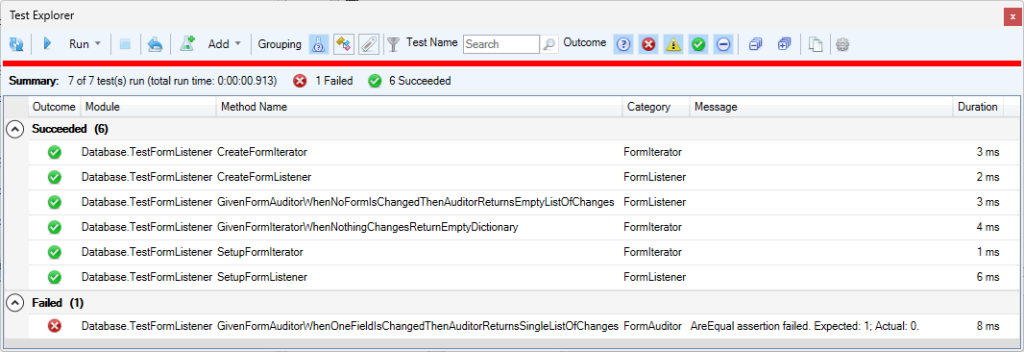
Nice, it even compiled, and of course we will want it to return one entry. So I’m going to try to implement the code to make this test pass as quickly as possible next time.